1. Components Basics
With great visual examples, this video covers: Introduction to Components, Props, Props Type checking, (This video example doesn't use SFC).
Intro to Vue.js: Components

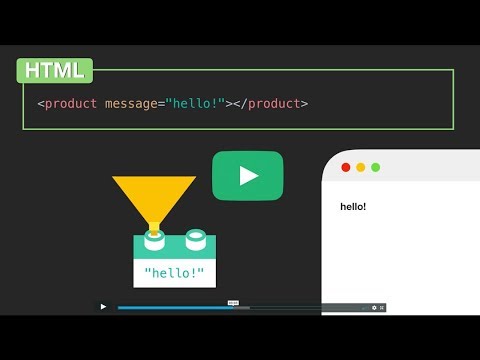
PS: The following article also talks about Component Props, which we will cover in the next section
An Introduction to Vue Components
2. Communication between components with Props and Emit
Vue.js creates a contract when communicating with parent/child components.
Pass data down to a child component
Props are used to pass data down to child components.
PS: This article also mentions Slots, which we will explore in more details later on.
Intro to Vue.js: Components, Props, and Slots | CSS-Tricks
Pass data up to a parent component
Now, when you want to communicate with a parent component, from the child, you can use the emit()
event.
3. Lifecycle hooks
@todo content
What are some real use-cases scenarios?
It's common to see the created
hook used to go and grab external data (from APIs, for example).
created () {
// fetch the data when the view is created
this.fetchData()
},
watch: {
// call again the method if the route changes
'$route': 'fetchData'
},
This video explores the following hooks:
created
,
mounted
,
updated
,
destroyed
and their
before*
variations.
Easy Way to Understand the VueJS Component Lifecycle Hooks

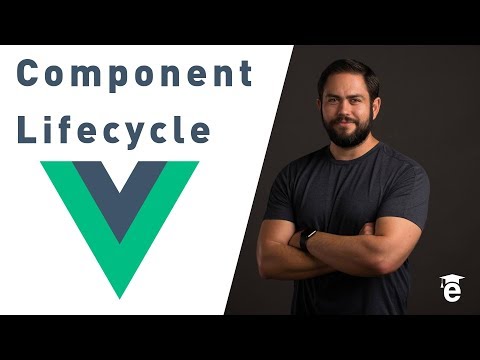
4. Single File Components
When creating a project using
vue-cli
, SFCs will be ready to be used as we've seen here @todo[link to 1st concept]. No additional configurations are required.
In this video, notice that the author uses
vue-cli
to create the project.
Components and .vue files | Learning the Basics

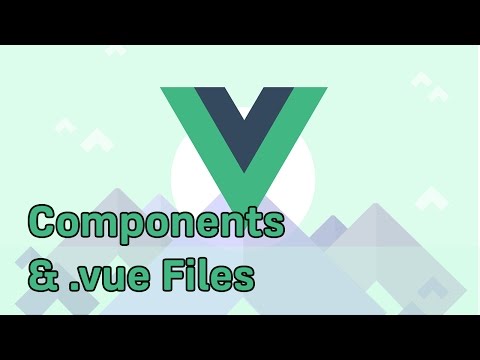
The official documentation explains really well all the benefits of using SFC files. This is the recommended approach and also how most Vue.js projects out there are structured:
Single File Components - Vue.js
What people are saying:
Sarah lists some great benefits of using SFC over separated html/css/js files:
A friend recently asked me this question: “what' s the benefit of using .vue files for single file components, vs creating a component with separate html/css/js files?”
— Sarah Drasner (@sarah_edo) February 27, 2018
This is a really good question! I' ll answer with my own opinion publicly in case it' s helpful (thread)
TL; DR
What does a SFC look like?
The basic structure of a single-file component is:
<template>
section<script>
section<style>
section
<template>
<div>
<!-- the root element doesn't necessarily need to be a div -->
</div>
</template>
<script>
export default {}
</script>
<style></style>
For example:
// TodoList.vue
<template>
<ul>
<li v-for="todo in todoList" :key="todo.id">{{ todo.name }}</li>
</ul>
</template>
<script>
export default {
data() {
return {
todoList: [
// an array of todo's should go here...
]
}
}
}
</script>
<style scoped>
li {
color: green;
}
</style>