1. Overview
Before jumping into the details, why not try to take a bird's eye view on how all the pieces and tools interact with each other?
⭐ Bob Ziroll created a 5 hours long full course for beginners
Learn React JS - Tutorial 2019

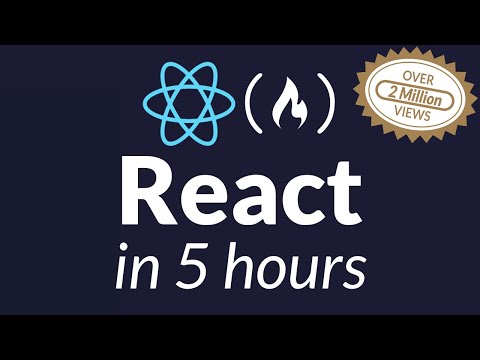
If 5 hours long is too much for you right now, you can study this video from Mosh, which is 2.5 hours long
React Crash Course [2019]

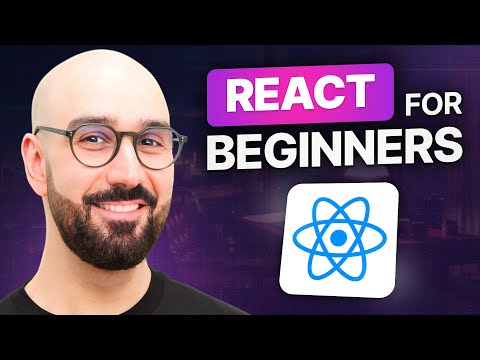
The official docs also recommend this article, from Tania Rascia, that covers "... essential React concepts and related terms, such as Babel, Webpack, JSX, components, props, state, and lifecycle":
Getting Started with React - An Overview and Walkthrough Tutorial
If you want to refresh your knowledge on JavaScript
JavaScript for React Developers | Mosh

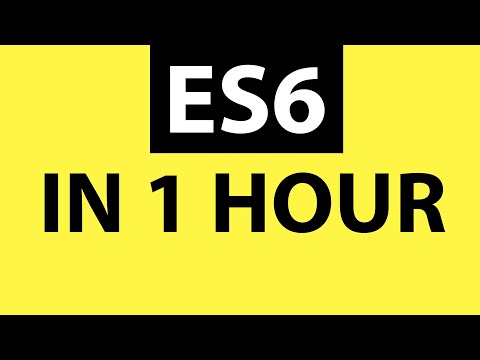
2. Installation
create-react-app
is an official React tool that lets you easily create your project and focus on the code straight away, without having to configure build tools.
Assuming you already have Node.js installed, run:
npm install -global create-react-app
Now, to create a new React app:
npx create-react-app project_name
# And you should see:
Installing packages. This might take a couple of minutes.
Installing react, react-dom, and react-scripts with cra-template...
yarn add v1.22.0
[1/4] Resolving packages...
[2/4] Fetching packages...
[#####--------------------------------------------------------------------------------------------------------] 59/1389
This will install and configure for you:
- A lightweight development server
- Webpack
- Babel
- Dependencies
3. Essential tools
4. Components, State and Props
Setting attributes
class MyComponent extends Component {
state = {
imageUrl: 'https://example/image.jpg'
imageAlt: 'An example image'
};
render() {
return (
<img src={this.state.imageUrl} alt={this.state.imageAlt}>
);
}
}
5. Writing HTML with JSX
Use .js or .jsx file extensions?
6. Working with Forms
@todo