1. Overview
JavaScript is one of the most popular programming languages in 2020. A lot of people are learning JavaScript to become front-end and/or back-end developers (using Node.js).
JavaScript is also the building-block of many front-end libraries/frameworks like React and Vue.js. Some developers jump straight into these frameworks and then realize they lack fundamental JavaScript concepts, so they take a step back and start studying JavaScript.
You'll have to go deeper on all these topics, but this is a must-watch if you're looking to get started, test your knowledge, or review.
JavaScript Tutorial for Beginners: Learn JavaScript in 1 Hour [2020]

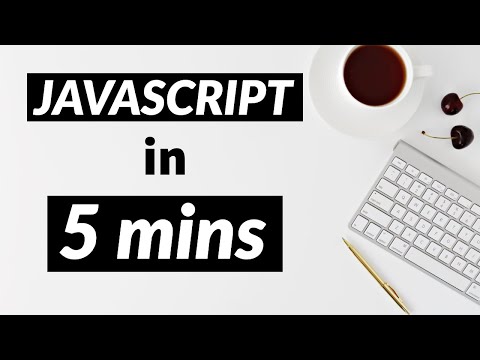
If you want to become a front-end developer, you have to learn JavaScript. It is the programming language that every front-end developer must know. I've designed this JavaScript tutorial for beginners to learn JavaScript from scratch. We'll start off by answering the frequently asked questions by beginners about JavaScript and shortly after we'll set up our development environment and start coding.
JavaScript Tutorial for Beginners: Learn JavaScript in 1 Hour [2020]
What is JavaScript · Variables · Constants · Primitive Types · Objects · Arrays · Functions

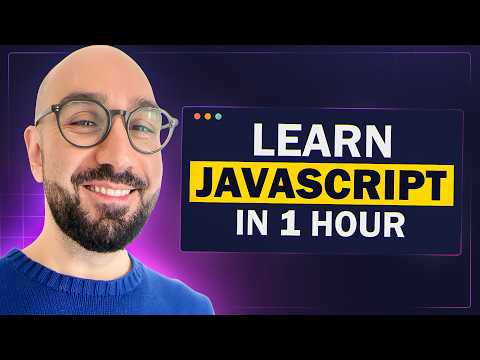
2. Objects
... in this modern JavaScript tutorial we'll take a deep dive into JavaScript objects. We'll look at object literal notation, how to make your own objects & some built in objects to the JavaScript language like the Math object.
Objects - Modern JavaScript Tutorial

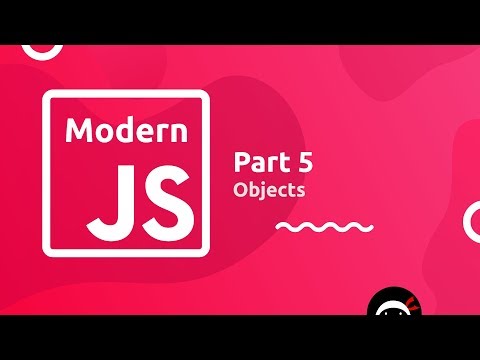
In this article, learn about: Properties and Methods, Accessing Object Properties, Adding and Modifying Object Properties, Removing Object Properties, Looping Through Object Properties.
Understanding Objects in JavaScript
3. Functions
A JavaScript function is a block of code designed to perform a particular task. For a function to be executed, something needs to invoke it (call it).
Basic example
// Example of a JavaScript function that greets a person
// 1. Declare/create the function
function greetUser(userName) {
// code to be executed
return 'Hi ' + userName + ', nice to have you here!'
}
// 2. Invoke the function, passing a parameter
greetUser('John')
// Data returned by the function:
"Hi John, nice to have you here!"
... in this modern JavaScript tutorial we'll take a look at functions - function declarations, function expressions, arrow functions and more...
Functions - Modern JavaScript Tutorial

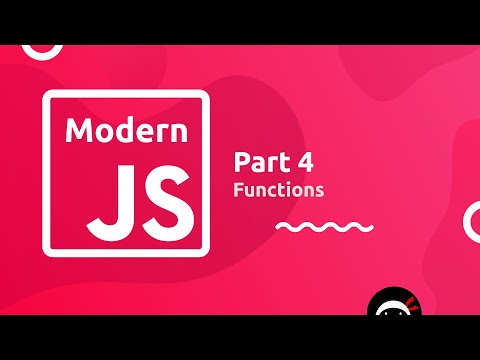
We cover how to create functions and use them to write clean and scoped code in JavaScript. After we are going to create a simple function that is going to take a string and uppercase it for us once we invoke the function.
Javascript Functions & Parameters | Javascript Tutorial For Beginners
Create a function · Different Types of Functions · Parameters

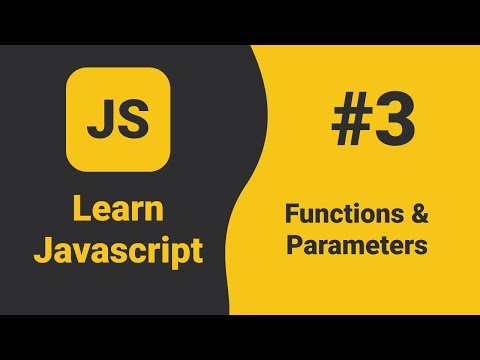
Functions are the main “building blocks” of the program. They allow the code to be called many times without repetition.
Functions
4. Arrays
Get familiar with Arrays and how to interact with them. Learn about the methods you can use to manage Array items, including adding, removing or finding a specific item from the Array.
Basic example
// Creating an Array
let fruits = ['Avocado', 'Apple', 'Banana']
// Acessing an Array item using the index position
fruits[0]
// Data returned:
"Avocado"
This video cover the basics of arrays and where to use them. It explains the usage of the pop()
method to remove a value from the end of the array, push()
method to add a value to the end of the array. Also covers other methods like shift()
and unshift()
.
Javascript Arrays | Javascript Tutorial For Beginners

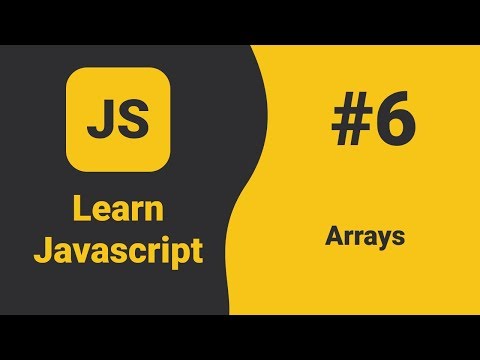
This article covers: Common operations, Accessing array elements, Array methods, length, index and more.
JavaScript Arrays - W3Schools
5. Loops and Iterations
Once you're acquainted with arrays, you can now start to explore loops and iterations! In computer programming, a loop is a sequence of instructions/statements that is repeated until a certain condition is reached.
In this video you will learn about all of the different types of loops in JavaScript. Loops are a way of repeating things in JavaScript. It will cover FOR, FOR.. IN, FOR.. OF, WHILE, DO.. WHILE, and the high order array function forEach.
JavaScript Loops Made Easy

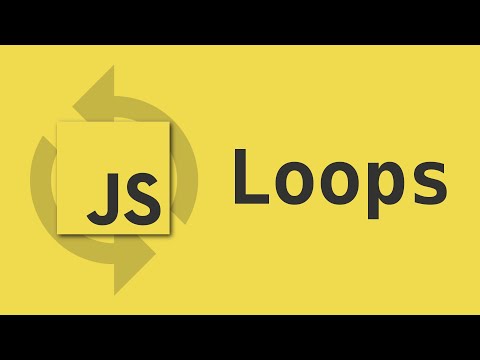
Programming loops are all to do with doing the same thing over and over again — which is termed iteration in programming speak.
Looping code
Loops are used in JavaScript to perform repeated tasks based on a condition. Conditions typically return true or false when analysed. A loop will continue running until the defined condition returns false.
JavaScript Loops Explained: For Loop, While Loop, Do...while Loop, and More
6. Scope
Though the concept of scope is not that easy to understand for many new developers, I will try my best to explain them to you in the simplest scope. Understanding scope will make your code stand out, reduce errors and help you make powerful design patterns with it.
Understanding Scope in JavaScript
Scope and context are not the same thing. Scope is variable access - what variables the current piece of code has access to, context is the value of
this
- when you typethis
in your code, what object is it referring to?This JavaScript tutorial covers scope and how it plays out in all of the functions that you create.
JavaScript Scope Tutorial

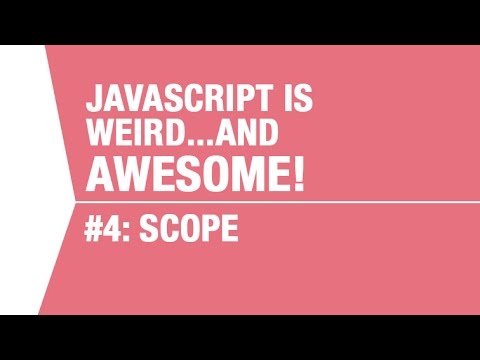
The scope is an important concept that manages the availability of variables. The scope is at the base closures, defines the idea of global and local variables.
If you’d like to code in JavaScript, understanding the scope of variables is a must.