1. Overview
This is a crash course of all the new features in JavaScript (ES6). We are going to take a look at all the new features, we will be writing all the code in old vanilla JavaScript and then we will refactor everything to ES6.
This course covers topics like arrow functions, the difference between a normal function and arrow functions, why not to use var and switch to const and let variables, object deconstruction, default parameters, switching from constructor functions to classes, promises and more.
ES6 JavaScript Tutorial For Beginners | ES6 Crash Course

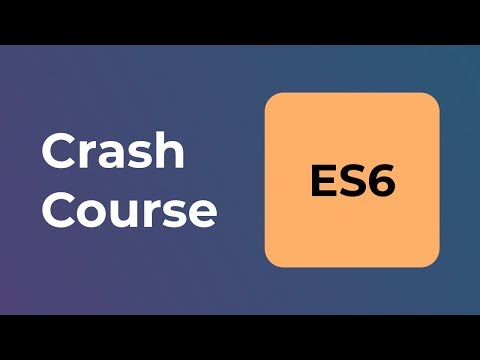
In the following course, learn over 16 concepts from the ES6+ world:
JavaScript is one of the most popular programming languages in the world. It’s used almost everywhere: from large-scale web applications to complex servers to mobile and IoT devices.
JavaScript ES6+: Learn to Code on the Bleeding Edge (Full Course)

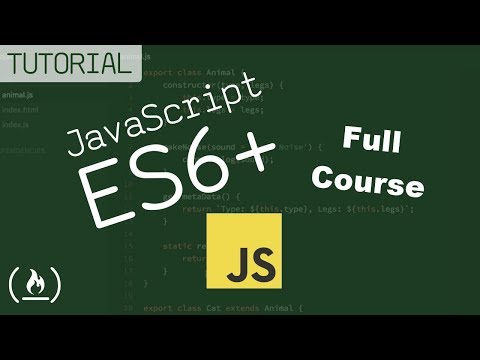
2. Arrow Functions
ES6 added many amazing new features to JavaScript, but by far one of the best features is arrow functions. Arrow functions not only make code much more concise and legible, but it also handles the scope of this in a much more intuitive way.
JavaScript ES6 Arrow Functions Tutorial

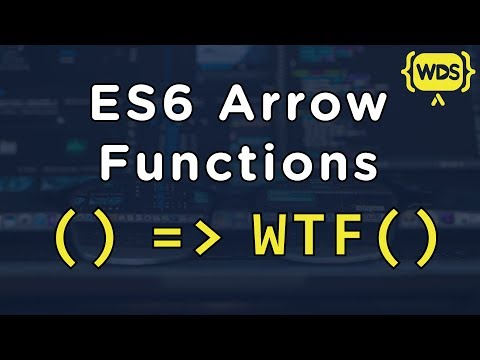
In this video I will explain arrow functions in JavaScript ES6. Arrow functions make your code shorter and more concise. They also handle the scoping of "this" in a more logical way than regular functions.
JavaScript ES6 Arrow Functions Tutorial

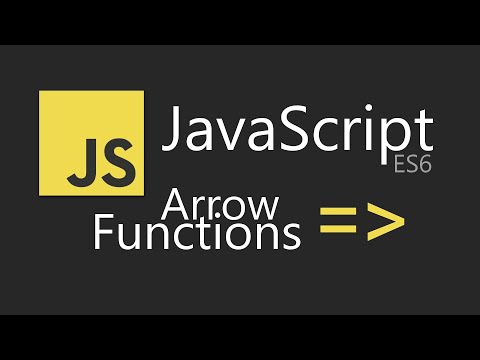
3. Promises
ES6 came with many new features, but one of the best features was the official introduction of Promises. Promises allow you to write clean non-callback-centric code without ever having to worry about callback hell. Even if you never write your own promise, knowing how they work is incredibly important, since many newer parts of the JavaScript API use promises instead of callbacks.
JavaScript Promises In 10 Minutes

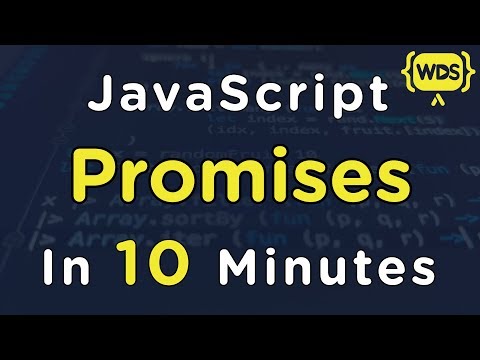
In this video, the author covers what promises are while creating an program that fetches a random word from an API and then uses this word to make another request what returns a random GIF related to that word.
Promises Part 1 - Topics of JavaScript/ES6

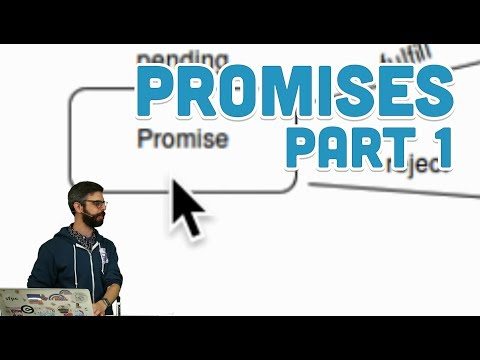
4. Async/Await
Learn how to make your JavaScript Promise code beautiful and concise with async-await.
The Async Await Episode I Promised

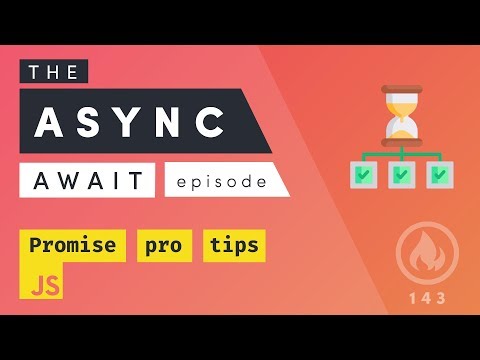
One of the hardest things about writing good JavaScript is dealing with heavily nested asynchronous code. Promises were created to solve the problem with callback hell, but there are still plenty of nested problems related to promises. This is where async/await comes in. JavaScript added async/await to allows developers to write asynchronous code in a way that looks and feels synchronous. This helps to remove many of the problems with nesting that promises have, and as a bonus can make asynchronous code much easier to read and write. In this video I am going to explain what async/await is and how to use async/await to rewrite your existing promise based code.
The Async Await Episode I Promised

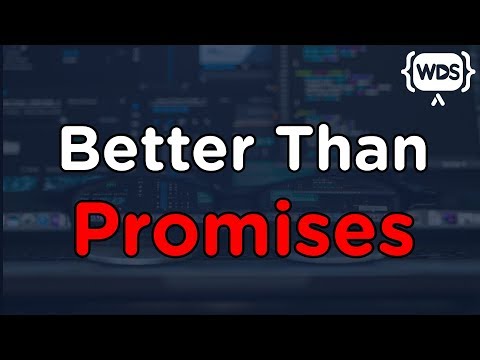
This video covers the new ES8 "async” and “await” keywords for writing asynchronous functions in JavaScript.
async/await Part 1 - Topics of JavaScript/ES8

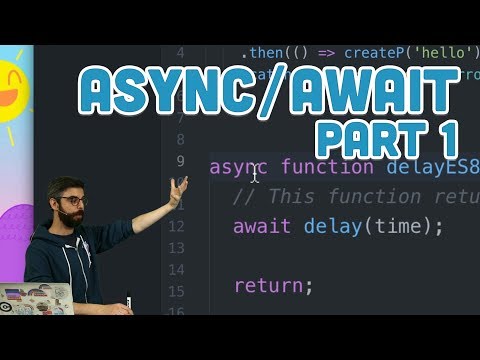
5. Template Literals
This is a really easy concept that won't take you more than 10 minutes to understand. It's very useful when you have multi-line strings or when you need to concatenate a string (aka. string interpolation).
We will cover how to do string concatenation in JavaScript with the "
+
" operator, we will also fix issues on when to use single or double quotes in strings.Finally we will take a look at the ES6 way of doing concatenations in JavaScript using the
${}
syntax.
JavaScript String Concatenation

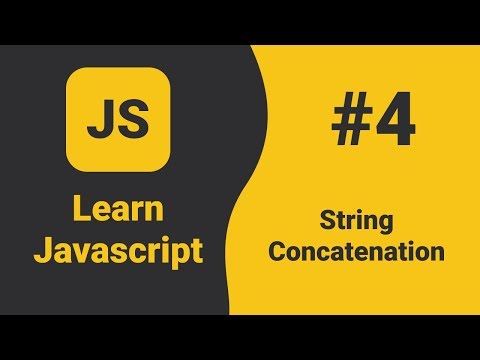
6. Destructuring
ES6 is one of the best things that ever happened to JavaScript. It added a ton of new features and syntax that made JavaScript so much more enjoyable to work with. One of the best features that ES6 added was object and array destructuring. With destructuring you can take apart an object or array incredibly easily. This has many use cases from making variable assignment much cleaner to the all important function argument destructuring. In this video I am going to show you exactly how destructuring works, and the best places to use destructuring.
Why Is Array/Object Destructuring So Useful And How To Use It

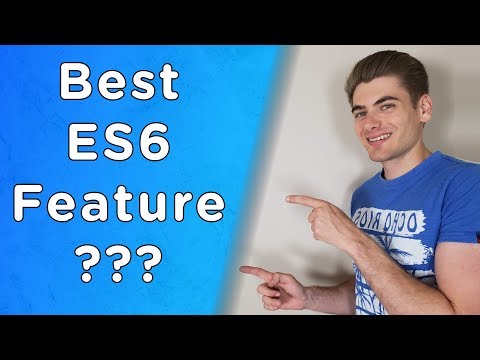
Destructuring assignment is special syntax for neatly assigning values taken directly from objects and arrays to variables. This is a new feature in JavaScript ES6.
Destructuring in ES6 - Beau teaches JavaScript

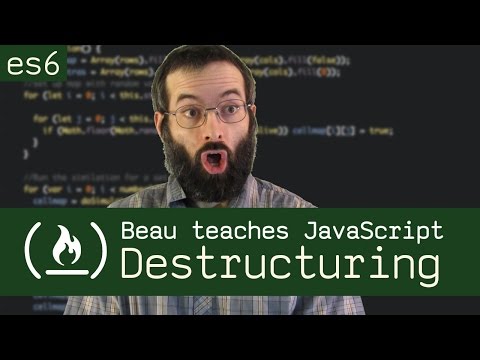